Neo-Pixel Ring Clock - ESP8266 Controlled
I purchased a 24 LED Neopixel Ring and was attempting to choose what to do with it. I had initially moved toward utilizing the ring for a Cheerlights extend however subsequent to making a Cheerlight out of it I concluded this was a horrible misuse of the ring. In this way, one end of the week when going by my neighborhood Makerspace (Medway Makers in the UK) I chose I would make a check out of it.
The ESP8266 is perfect for this as has wifi so the time can be gotten over WiFi and it can likewise control the Neopixel Ring. I utilize the Wemos D1 Mini as they are extremely easy to utilize, have a miniaturized scale USB association for power and information, cost just a couple bucks and can be utilized effortlessly with the Arduino IDE. This is an extremely basic venture with basic code and very little in the method for a circuit.
The Neopixel ring has 3 associations: 5v, Ground and Data. You should simply to weld 3 wires to these 3 cushions on the ring. VCC and Gnd go to 5v and Ground on your D1 Mini and the Data stick can go to any yield stick.
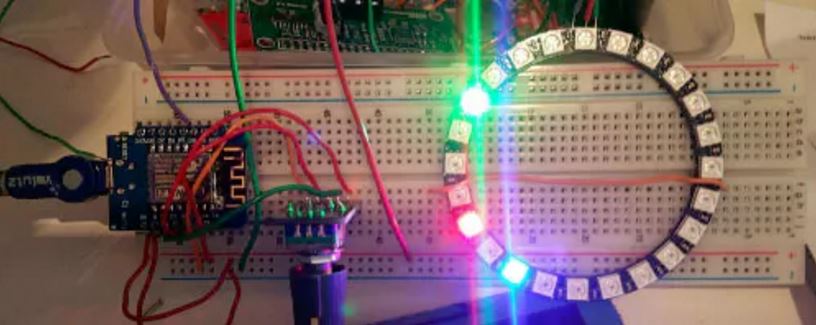
The Code Requires 3 libraries:
#include <Adafruit_NeoPixel.h>
#include <ESP8266WiFi.h>
#include "TimeClient.h"
The Adafruit_NeoPixel library can be downloaded here
The TimeClient library is part of the Squix Weather Station code and can be downloaded here
Just copy the TimeClient.cpp and TimeClient.h files into your sketch folder.
The TimeClient library works by interfacing with the web by means of WiFi and going to www.google.com. It then scratches the header of the website page to acquire the time and date from the header.
The ESP8266WiFi library accompany the Arduino IDE when you introduce the ESP8266 as a perceived board. Bear in mind to introduce the ESP8266 documents utilizing the Arduino IDE Boards Manager before you begin your venture.
We will utilize Pin 5 on the D1 Mini for the information line.
#define PIN D5
We have to monitor, in our code, when the last overhaul of the time from the web happens furthermore to what extent it has been since one moment back (to monitor seconds).
long lastUpdate = millis();
long lastSecond = millis();
The hours, minutes and seconds are stored in a String variable.
String hours, minutes, seconds;
As well as the current second, minute and hour which we keep track of.
int currentSecond, currentMinute, currentHour;
Character arrays are used to store your WiFi SSID and Password. Enter your own details here:
char ssid[] = "xxxxxxx"; // your network SSID (name)
char pass[] = "xxxxxxx"; // your network password
I live in London so I have 0 hours offset from UTC, except in Summer Time when it will be 1 hour. Set the offset for your own time zone.
const float UTC_OFFSET = 0;
We create a timeClient object and pass it the UTC offset.
TimeClient timeClient(UTC_OFFSET);
Then create a NeoPixel object, call it 'strip' and pass it the number of LED's and what pin we are using for the data line.
Adafruit_NeoPixel strip = Adafruit_NeoPixel(24, PIN);
In the setup function we will start off by opening serial comms for debug purposes.
void setup()
{
Serial.begin(115200);
Then the NeoPixel ring needs to be initialised and we set brightness to 50%
strip.setBrightness(128);
Nothing happens on the ring until we do a .show command.
strip.show();
Next we connect to your WiFi
WiFi.begin(ssid, pass);
while (WiFi.status() != WL_CONNECTED)
{...
Then update the time from the timeClient library.
timeClient.updateTime();
Next we run our own function called updateTime(); which calls the library functions to get the current hours, minutes and seconds.
updateTime();
At long last, before the fundamental circle, we recover and store the present estimation of millis() so we can track of to what extent it has been since we last redesigned the time from the web furthermore monitor track of the seconds as they go by so the second "hand" can be overhauled.
lastUpdate = millis();
lastSecond = millis();
The setup function is complete. Next the main loop function will run.
void loop()
If 1,800,000 milliseconds (30 minutes) have passed by since we last updated the time, we update the time again from the internet.
if ((millis() - lastUpdate) > 1800000) updateTime();
The hours, minutes and seconds are updated on the display every second so we need to check if 1000 milliseconds have passed since we last updated the display. If so the code within the if statement is carried out.
if ((millis() - lastSecond) > 1000)
The colours of the 'hands' are:
- RED = Hours
- GREEN = Minutes
- BLUE = Seconds
The .setPixelColor command is utilized to set the shades of the 'hands'. The ring I utilized had 24 RGB LED's so the seconds and minutes get separated by 2.5 so they are shown on the right quadrant of the ring, the hour (24 hour arrange) gets isolated by 2. Prior to the time is transformed we set the present "hands" to OFF to clear the last positions.
strip.setPixelColor(currentSecond / 2.5, 0, 0, 0);
strip.setPixelColor(currentMinute / 2.5, 0, 0, 0);
strip.setPixelColor(currentHour * 2, 0, 0, 0);
strip.show();
The current value of millis() is now stored.
lastSecond = millis();
Next, the value put away in currentSecond is expanded by 1. We then check if the seconds have gone more than 59 and if so set them back to 0. At the point when that happens we additionally increment the minutes by 1 and do a similar check. In the event that the minutes go more than 59 then we likewise increment the hour by 1.
currentSecond++;
if (currentSecond > 59)
{ currentSecond = 0;
currentMinute++;
if (currentMinute > 59) {
currentMinute = 0;
currentHour++;
if (currentHour > 12) currentHour = 0;
}
}
Although not necessary, I print out the current time to the serial monitor window for debugging purposes. I use a String object to generate the time string.
String currentTime = String(currentHour) + ':'
+ String(currentMinute) + ':' + String(currentSecond);
Serial.println(currentTime);
Since the seconds, minutes and hours have been upgraded we can set the important "hands" to their separate RGB values.
strip.setPixelColor(currentSecond / 2.5, 0, 0, 255);
strip.setPixelColor(currentMinute / 2.5, 0, 255, 0);
strip.setPixelColor(currentHour * 2, 255, 0, 0);
strip.show();
At last we make our own particular capacity called updateTime(). This gets overhauled toward the begin and after that again like clockwork after to keep exact time.
void updateTime();
{
We obtain the hours, minutes and seconds from the timeClient library.
hours = timeClient.getHours();
minutes = timeClient.getMinutes();
seconds = timeClient.getSeconds();
and store those values as integers (They are returned as strings from the timeClient library). 24 hours are converted to 12 hour format.
currentHour = hours.toInt();
if (currentHour > 12) currentHour = currentHour - 12;
currentMinute = minutes.toInt();
currentSecond = seconds.toInt();
Finally, we want to be able to check that 30 mins have passed since the last update so we store the current value of millis() again.
lastUpdate = millis();
My clock is presently only a model on a breadboard, as should be obvious in the picture. Be that as it may, this would look incredible underneath some scratched white acrylic so I provoke you to utilize the code above to make your own particular ring clock and make something delightful out of it.
Content edited by HackerSpaceTech as granted by CC BY does not carry any endorsement from original author
Mike McRoberts
Published
Thu 08 December 2016
Comments
comments powered by Disqus